Introduction
Welcome to an in-depth exploration of the Java Calendar API, a powerful toolkit for handling dates and times in Java applications. This comprehensive tutorial aims to provide a thorough understanding of the Java Calendar class and its versatile capabilities. In this tutorial, we will explore essential methods of the Java Calendar class with practical examples to help you understand how to manipulate dates and times in your Java programs. Let’s dive in!
Foundations of the Calendar Class:
- The Calendar class in Java has an abstract nature, acting like a master organizer for date and time activities.
- It’s designed to simplify complex tasks related to handling dates and times in Java, making your code more efficient.
- Think of the Calendar class as your date translator. It smoothly converts between different moments in time.
- Its key role includes managing essential calendar details like the year, month, and day, providing a versatile tool for time-related operations.
Creating Calendar Instances:
Instantiating the abstract Calendar class is achieved through the static method Calendar.getInstance()
.
Calendar calendar = Calendar.getInstance();
Customizing Calendar Instances: Calendar instances can be customized by specifying time zones or locales, providing flexibility.
// Specify a time zone
Calendar calendarWithTimeZone = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
// Specify a locale
Calendar calendarWithLocale = Calendar.getInstance(Locale.US);
// Specify both time zone and locale
Calendar customCalendar = Calendar.getInstance(TimeZone.getTimeZone("GMT"), Locale.US);
Working with Calendar Fields: Access and manipulate specific fields like year, month, and day.
int year = calendar.get(Calendar.YEAR);
int month = calendar.get(Calendar.MONTH); // Note: Months are zero-based (0 - 11)
int day = calendar.get(Calendar.DAY_OF_MONTH);
import java.util.Calendar;
import java.util.TimeZone;
public class CalendarExample {
public static void main(String[] args) {
Calendar calendar = Calendar.getInstance(TimeZone.getTimeZone("GMT"));
int year = calendar.get(Calendar.YEAR);
System.out.println("Current year : " + year);
int month = calendar.get(Calendar.MONTH); // Note: Months are zero-based (0 - 11)
System.out.println("Current month : " + month);
int day = calendar.get(Calendar.DAY_OF_MONTH);
System.out.println("Current day : " + day);
}
}
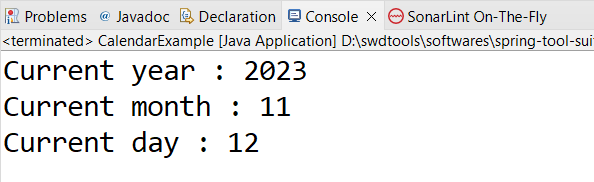
Modifying Calendar Values Dynamically: Modify specific fields or add/subtract values using methods like set()
and add()
.
// Set the year to 2023
calendar.set(Calendar.YEAR, 2023);
// Add one month to the current date
calendar.add(Calendar.MONTH, 1);
Comparing Calendar Instances: Utilize the Comparable
interface to compare Calendar instances.
Calendar anotherCalendar = Calendar.getInstance();
if (calendar.compareTo(anotherCalendar) > 0) {
// calendar is after anotherCalendar
}
Conclusion
The Java Calendar API proves to be an invaluable resource for managing temporal aspects in Java applications. This tutorial equips you with the foundational knowledge to navigate, customize, and manipulate dates and times effectively. As you explore the details of the Java Calendar class, you’ll get better at dealing with dates and times in your code. Happy coding!
2 thoughts on “Java Calendar API”
Comments are closed.
Informative.
Informative