There are several ways in which we can create java objects. In this post, we will have a look at some of these.
1. Using new keyword
We have used this approach mostly to create objects in java. This is the most common and basic way. In this way, we call constructor with parameters or with no arguments. The new keyword allows us to create a new object.
Doctor d = new Doctor();
Cricketer c1 = new Cricketer(1, 'Virat Kohli');
Animal a = new Animal();
2. Using NewInstance method of Class class
In this way, a no-argument constructor is called by the newInstance() method to create an object. The newInstance() method is a reflective way to create an object since it belongs to java.lang.reflect.Constructor class.
The Class.forName() is used to load the class dynamically. The newInstance() method uses the following syntax:
public T newInstance() throws InstantiationException,IllegalAcccessException
Note -> If the method or class is not accessible, it returns the IllegalAccessException.
Note -> If the Class represents a primitive data type, an abstract class, an interface, an array class, or if the class does not have a no-arg constructor, it returns an InstantiationException message.
package com.javatrainingschool;
public class NewInstanceExample
{
String str = "Hello Java";
public static void main(String[] args) {
try{
Class cls = Class.forName("NewInstanceExample");
NewInstanceExample newObj = (NewInstanceExample) cls.newInstance();
System.out.println(cls.str);
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (InstantiationException ex){
ex.printStackTrace();
} catch (IllegalAccessException ex) {
ex.printStackTrace();
}
}
}
3. Using newInstance() method of Constructor class
public T newInstance(Objects...initargs)
The following exceptions are returned by the newInstance() method:
- Incase the constructor cannot be accessed, the method throws IllegalAccessException.
- If the number in the formal and actual parameters differ, it throws IllegalArgumentException.
- If an exception is thrown by the constructor, it throws InvocationTargetException.
If the initialization fails, it throws ExceptionInInitializerError.
import java.lang.reflect.Constructor;
import java.lang.reflect.Constructor;
public class NewInstanceExample {
String str="Hello World";
public static void main(String args[]) {
try{
Constructor<NewInstanceExample> cons = NewInstanceExample.class.getConstructor();
NewInstanceExample newObj = cons.newInstance();
System.out.println(newObj.str);
}catch(Exception ex) {
ex.printStackTrace();
}
}
}
4. Using serialization/ deserialization
Serialization persists the state of an object. Deserialization creates the object from that persisted state. Below example illustrates how we are serializing and deserializing object of Doctor class
package com.javatrainingschool;
import java.io.Serializable;
//Class must implement Serializable interface
//which is a marker interface
public class Doctor implements Serializable {
/**
*
*/
private static final long serialVersionUID = 1L;
private int id;
private String name;
private String department;
//constructors, getter/setters, and tostring()
}
Class that serializes the object
package com.javatrainingschool;
import java.io.FileOutputStream;
import java.io.FilterOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
public class SerializationExampleClass {
public static void main(String[] args) throws IOException {
Doctor doctor = new Doctor(1, "Dr Ajay", "Cardiology");
String fileName = "C:\\Users\\abc\\Desktop\\Training\\abc.txt";
FileOutputStream fout = new FileOutputStream(fileName);
ObjectOutputStream out = new ObjectOutputStream(fout);
out.writeObject(doctor);
out.close();
fout.close();
System.out.println("The object doctor has been saved successfully.");
}
}
The class that deserializes the object
package com.javatrainingschool;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.ObjectInputStream;
public class DeSerializationExample {
public static void main(String[] args) throws IOException, ClassNotFoundException {
String fileName = "C:\\Users\\abc\\Desktop\\Training\\abc.txt";
FileInputStream fin = new FileInputStream(fileName);
ObjectInputStream in = new ObjectInputStream(fin);
//deserialize the object
Doctor d = (Doctor)in.readObject();
System.out.println("The state of doctor : " + d);
in.close();
fin.close();
}
}
5. Using clone() method
We can create object of a clonable class by calling clone() method.
Note -> We can clone object of only those classes that implements Clonable interface.
Note -> Clonable is a marker interface
package com.javatrainingschool;
public class Car implements Cloneable {
private String name;
private String type;
public Object clone()throws CloneNotSupportedException{
return super.clone();
}
//getter and setters
//constructors
//toString
}
package com.javatrainingschool;
public class CloningExample {
public static void main(String args[]){
try{
Car c1 = new Car("BMW 5 Series", "Sedan");
Car c2 = (Car)c1.clone(); //cloning the c1 object
c2.setName("BMW 5 Series Cloned Version");
System.out.println("Original Car : " + c1); //prints info of the original object
System.out.println("Cloned Car : " + c2); //prints info of the cloned object
}
catch(CloneNotSupportedException ex){
ex.printStackTrace();
}
}
}
Conclusion
In this post we saw that ‘new’ is not the only way to create object of a class. There are other ways also. Now, this is time to get our Coffee object. Let’s enjoy
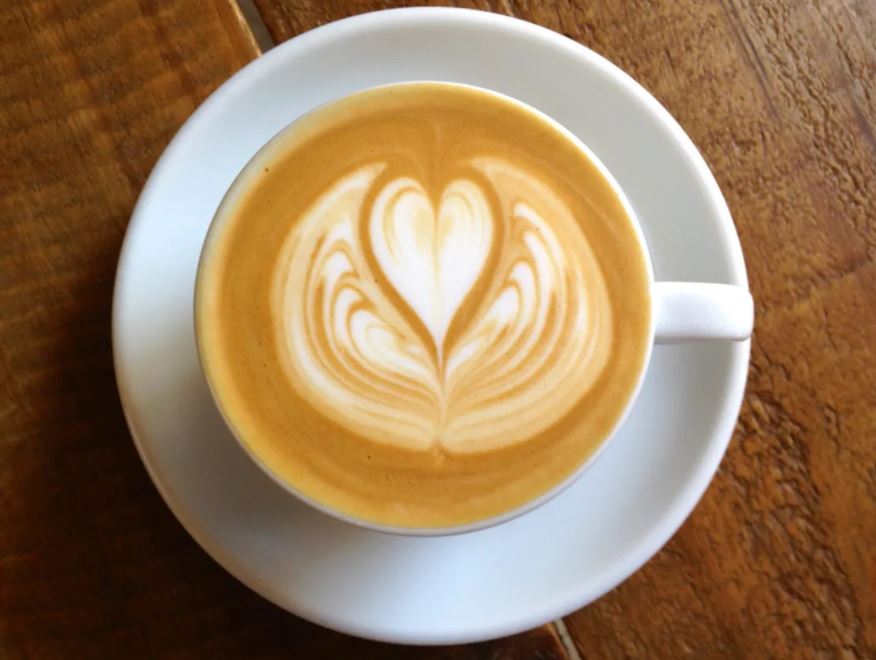