Java is strictly pass-by-value. Meaning when we call a method, instead of the actual reference variable, a copy of it is sent to the method. Let’s consider below example
public class PassByValueExample {
public static void main(String [] args) {
int val = 10;
System.out.println("Before manipulation val : " + val);
int result = manipulateValue(val);
System.out.println("After manipulation val : " + val);
System.out.println("Result : " + result);
}
public static int manipulateValue(int val) {
val = 20;
return val;
}
}
Output :
Before manipulation val : 10
After manipulation val : 10
Result : 20
You can be ascertain that the original value of variable val is still unaffected. This happens because local variable val in the method manipulateValue is a copy of the passed variable val from main method. Let’s understand from the below diagram.
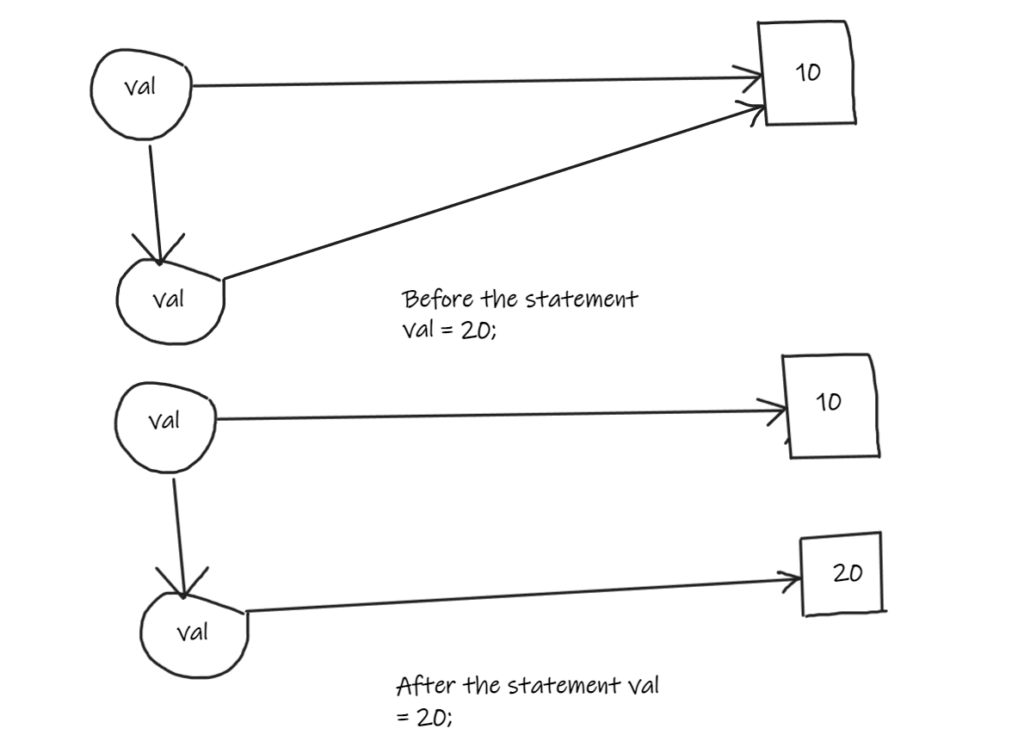
When the statement is written val = 20; inside manipulateValue() method, method’s local variable starts poiting to new primitive int value 20. And the value referenced by main method’s variable val remains unchanged.
Pass-by-value in case of objects
Let’s consider below example.
public class Book {
int id;
String name;
public Book(int id, String name) {
super();
this.id = id;
this.name = name;
}
public Book() {
super();
// TODO Auto-generated constructor stub
}
}
Scenario One : In this scenario, we are assinging a new book object to the local variable book in changeBookInfo method. So, whatever changes we make in this object will not affect the original object that is being passed in the main method.
public class BookMain {
public static void main(String[] args) {
Book b = new Book(10, "Let us Learn Java");
changeBookInfo(b);
System.out.println("Id : " + b.id);
System.out.println("book : " + b.name);
}
public static Book changeBookInfo(Book book) {
book = new Book();
book.id = 20;
book.name = "Let us C";
return book;
}
}
In the below output, you can see that despite the fact that we are chaning book info in changeBookInfo method, passed book object’s values don’t change. Because of the fact that we are only passing the copy of reference to the method.
Output :
Id : 10
book : Let us Learn Java
Scenario 2 : If we don’t assign a new book object inside changeBookInfo method, local variable book will still point to the object referred by the original variable in the main method. And thus any changes made in the changeBookInfo method will also be seen by the main method original book reference variable.
public class BookMain {
public static void main(String[] args) {
Book b = new Book(10, "Let us Learn Java");
changeBookInfo(b);
System.out.println("Id : " + b.id);
System.out.println("book : " + b.name);
}
public static Book changeBookInfo(Book book) {
book.id = 20;
book.name = "Let us C";
return book;
}
}
Output :
Id : 20
book : Let us C
Conclusion
It is clear from the above examples that java is pass-by-value. So, we can conclude that our original reference variable remains unaffected due to this feature of java.