Recursion is a technique in which a method keeps on making calls to itself until some break condition occurs. We can use this technique in the scenarios where we have to perform similar task repeatedly for a certain number of times. In this post, we will use recursion technique to reverse a string.
This is how the String will be reversed
- First pick the letter at the last index of the String
- Append this letter to a StringBuffer object and remove this letter from the original String
- Call the same method again with the updated String in step 2
- When there is only one letter remaining, simply append the it to the StringBuffer object and break the recursive call.
Interesting Fact -> We all know the legendary Bollywood singer Kishor Kumar. There is an interesting fact about him. Often, upon being asked his name, he used to tell his name in reverse order of alphabets. So, let’s see what he used to say by the running the below program that reserses a string.
Let’s understand the recursion from the diagram
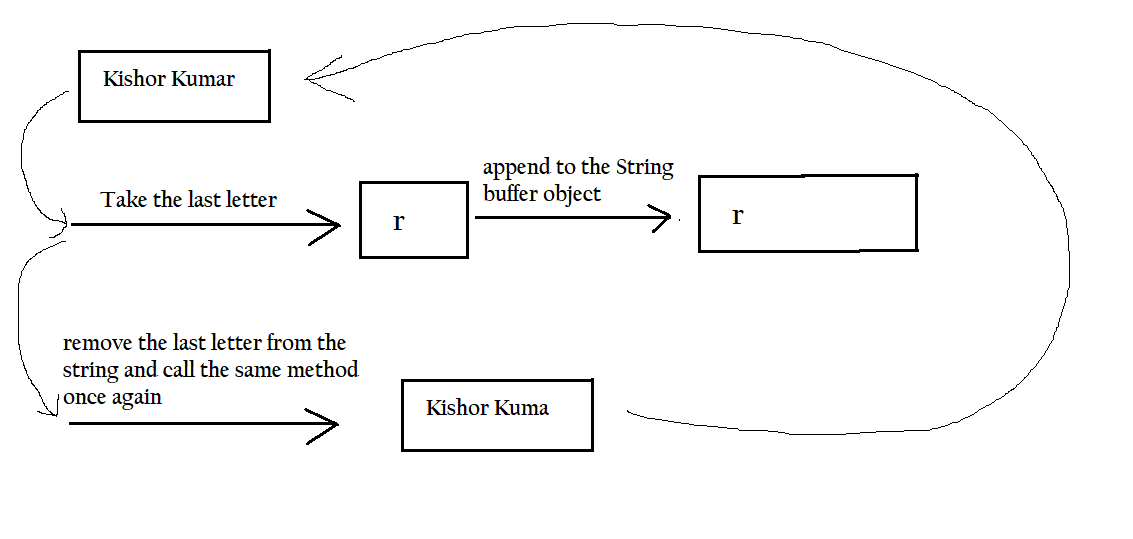
package com.javatrainingschool;
public class StringReversalUsingRecursion {
private static StringBuffer reversedName = new StringBuffer();
public static String reverseStringM(String name) {
if (name == null || name.length() <= 1) {
reversedName.append(name);
} else {
reversedName.append(String.valueOf(name.charAt(name.length() - 1)));
reverseStringM(name.substring(0, name.length() - 1));
}
return reversedName.toString();
}
public static void main(String[] args) {
String name = "Kishor Kumar";
System.out.println("Original name : " + name);
System.out.println("Reversed Name : " + reverseStringM(name));
}
}
Output :
Original name : Kishor Kumar
Reversed Name : ramuK rohsiK